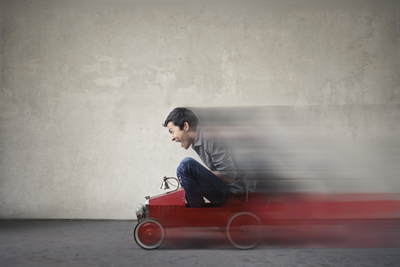
Introduction
Ref structs are a type of struct in C# that provides stack-based allocation and deallocation. They offer significant benefits such as reduced memory consumption, eliminated garbage collection overheads, and improved performance.
What is a Ref Struct?
A ref struct is a type of struct that is allocated on the stack rather than on the heap. This means that instances of a ref struct are automatically deallocated when they go out of scope, eliminating the need for garbage collection.
Benefits of Ref Structs
Ref structs offer numerous benefits, including:
- Reduced Memory Consumption: Ref structs eliminate the need for heap allocation, reducing memory consumption and improving performance.
- Eliminated Garbage Collection Overheads: With ref structs, you don't need to worry about garbage collection overheads, making your application more efficient.
- Improved Performance: Ref structs are faster than their heap-allocated counterparts since they avoid the overhead of garbage collection.
Limitsations of Ref Structs
While ref structs offer many benefits, there are some limitations to be aware of:
- Cannot Be an Element of an Array: Ref structs cannot be elements of arrays.
- Cannot Be Boxed: Ref structs cannot be boxed to `System.Object` or `System.ValueType`.
- Cannot Be a Member of a Struct: A ref struct cannot be a member of another struct.
- Cannot Be Used as a Generic Argument: Ref structs cannot be used as generic arguments when calling methods.
- Cannot Be Captured in a Local Function or Lambda Expression: Ref structs cannot be captured in local functions or lambda expressions.
- Must Implement All Interface Members: When implementing an interface, a ref struct must implement all interface members.
Best Practices
To get the most out of ref structs, follow these best practices:
- Use Ref Structs for Temporary Objects: Use ref structs to create temporary objects that are automatically deallocated when they go out of scope.
- Avoid Overusing Ref Structs: Be careful not to overuse ref structs in your application, as excessive usage can lead to performance issues.
- Implement the Dispose Pattern: Implement the dispose pattern using a ref struct to ensure proper resource release.
Example Code
Here's an example code snippet demonstrating the use of a ref struct:
public ref struct MyRefStruct { public void Dispose() { /* Release resources */ } } public class MyClass { public void MyMethod() { MyRefStruct myRefStruct = new MyRefStruct(); // Use myRefStruct within the scope of MyMethod() } }
Conclusion
Ref structs are a powerful tool for reducing memory consumption and eliminating garbage collection overheads. By understanding their benefits, limitations, and best practices, you can effectively use ref structs in your C# applications to improve performance and efficiency.